JS++ now supports the ‘class’ keyword. As promised, we would have classes available by Q1 2017. Notably, we are providing “basic” classes, so the following features are supported:
- ‘class’ keyword
- Inheritance
- Constructors (including private/protected constructors that limit instantiation/inheritance)
- Static Constructors
- Instantiation
- Fields
- Methods
- ‘this’ keyword
- ‘super’ keyword
- Method Overloading
- Constructor Overloading
- Getters and Setters (via ‘property’ keyword)
- Type system support
- Debugger support for classes (via source maps)
What did we consider to be outside the scope of basic classes? The following features are currently not available yet:
As an example of what you can do with JS++ classes, we included an example with the Tiled Map Editor:
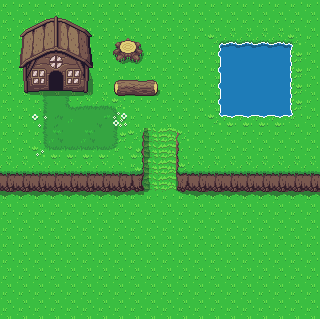
The included sample for Tiled can be found in the ‘Samples/Classes/tiled-loader’ directory with JS++ 0.5.0. Currently, it will load all maps exported to JSON with orthogonal tiles. It’s just a small but powerful example of what you can start doing with JS++ for early adopters.
I am also happy to inform that the backend for the JS++ website is written completely in JS++, and it has now run stable for one week without a single crash or error (other than 404 errors).
Finally, we have made the decision to not include the Mac/Linux installer… yet.
Be sure to check out the documentation on the ‘class’ keyword to get up to speed on JS++ classes.
Download JS++ 0.5.0 from our home page.