JS++ does not implement a const
keyword. This was a design decision.
For instance, consider the following ECMAScript 6 code:
const x = {};
// Adding a new property, not "constant" at all
x.foo = 1;
// Changing the value of an existing property; once again, not "constant"
x.foo = 2;
x.foo; // 2
The variable x
is declared as a constant using the const
keyword. However, it very clearly is not constant (e.g. mathematical constants, which are typically our first exposure to constants). Where references are concerned, const
does not actually create a “constant” and should thus be regarded as either a misnomer, and, in a worst case, should be disallowed as a matter of best practice in order to discourage semantic confusion and – consequently – bugs.
The situation is bad enough that Brendan Eich, the creator of JavaScript, even retweeted a blog post about this confusion:
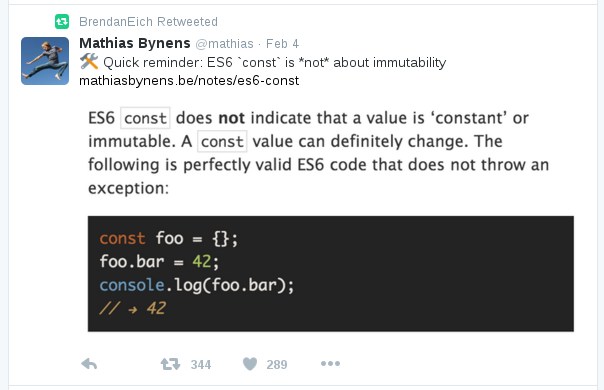
Instead, JS++ uses the final
keyword. final
prevents any further assignments to a variable; in other words, this is the last and “final” assignment. Thus, while the reference can be modified, the actual variable is still referring to that same reference. It was a final reference, and the reference we were referring to cannot be changed. Simple semantics and functionally identical, but it can go a long way in complex projects.
You never know if you’ll have a team member that doesn’t know the nuances of const
, and – going back to semantic confusion – naturally assumes it works a certain way without looking it up and researching it. This is a common problem with JavaScript, where a lot of developers assumed it would be like C/C++ or Java and, thus, did not spend too much (if any) time learning it properly. Personally, I was guilty of much of the same in my early days. const
being introduced into the official language specification did not take this behavioral issue into account and risks exacerbating the problem. (ASIDE: Issues like this, while minor and seemingly innocuous on the surface, can create exponentially many problems in larger applications and forced the hand for JS++ to break away from the ECMAScript specification – among a host of other problems.)
Yes, languages like C++ get away with using const
. However, it can be argued that it’s so deeply embedded into the language in special ways it almost forces programmers to read the documentation and learn about it in depth (see: “const correctness” in C++). When you see:
const int *const ptr = &x;
You begin to realize that there is more to const
in C++ than you may have figured out through a pure dictionary definition. This is not so when you see this:
const x = {};
The latter example (in JavaScript) does not encourage you to read the documentation or best practices. When we make assumptions that we “just know,” it can be dangerous.
We made a design decision based on experience and observation in the hope that final
will be better because it doesn’t necessarily encourage the “I just know what it does” attitude. When it does, they should already be familiar with how it works from Java – a correct, consistent, non-confusing, and – most importantly – safe expectation.